File Handling in C
3 min read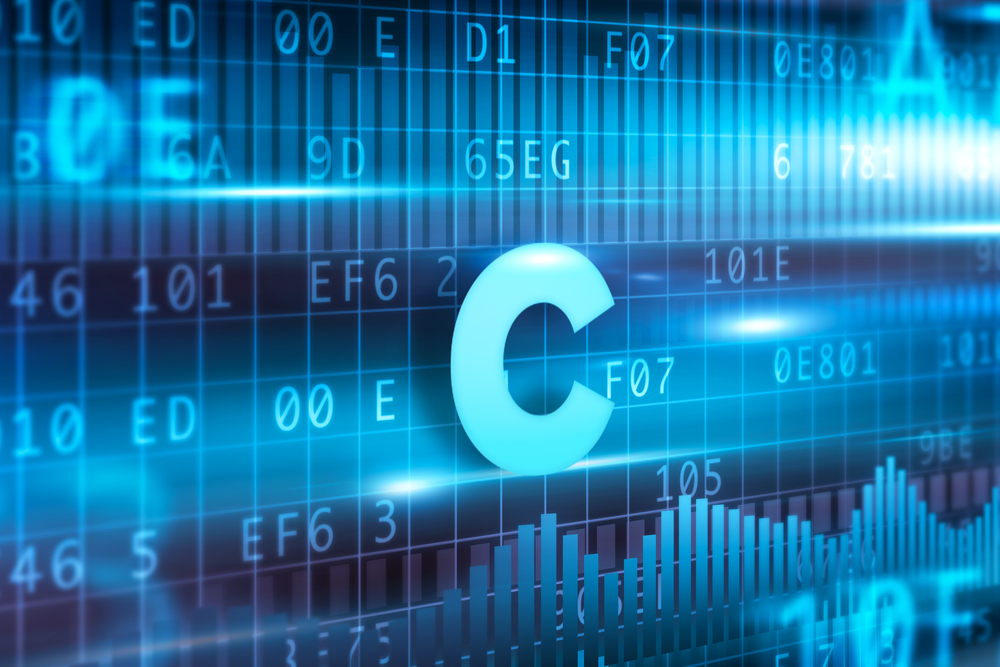
When programming, one might need to generate some specific input multiple times. Thus, sometimes it might not be enough to display the data only on the console. This data to be displayed may even be very large. As a result, only a limited data amount can be displayed on the console. Also, since the memory here is volatile, it is impossible for us to recover the data generated programmatically again and again. But, if we want to do so, then we may store it on the local file system. It is volatile and we can access it every time. It is exactly where we need file handling in C.
In C language, file handling enables us to update, create, read, and even delete the files that we may have stored on the local file system via our C program. As a result, we can perform the following operations on a file:
- Deleting a file
- Opening an existing file
- Creating a new file
- Reading from a file
- Writing to a file
Functions for file handling
Many functions can be used in the C library to read, open, write, close and search the file. Here is a list of file functions used in a program:
Function | Description |
rewind() | sets the file pointer to the beginning of the file |
fprintf() | write data into the file |
fopen() | opens new or existing file |
fputc() | writes a character into the file |
fscanf() | reads data from the file |
fclose() | closes the file |
fgetc() | reads a character from file |
fputw() | writes an integer to file |
fseek() | sets the file pointer to given position |
ftell() | returns current position |
fgetw() | reads an integer from file |
Opening File: fopen()
One must open a file before reading, writing, or updating it. We use the fopen() function when we want to open a file. The syntax of the fopen() is:
FILE *fopen( const char * filename, const char * mode );
The fopen() function would accept two parameters:
- The mode in which a file is to be opened. It is referred to as a string.
- The string (file name). In case the file is stored at any specific location, we have to mention the path at which this file is actually stored. For instance, a file name could be like “c://some_folder/some_file.ext”.
Also Read: Various Benefits of education
One can use one of these modes in the function fopen().
Mode | Description |
w | opens a text file in write mode |
r | opens a text file in read mode |
a | opens a text file in append mode |
a+ | opens a text file in read and write mode |
ab | opens a binary file in append mode |
ab+ | opens a binary file in read and write mode |
w+ | opens a text file in read and write mode |
wb | opens a binary file in write mode |
r+ | opens a text file in read and write mode |
rb | opens a binary file in read mode |
rb+ | opens a binary file in read and write mode |
wb+ | opens a binary file in read and write mode |
The function fopen() works in the following way:
- It searches for the file to be opened.
- It loads the file from a disk and then places it into the buffer. We use the buffer to provide efficiency for any read operations.
- It then sets up a character pointer that helps us point to the very first character of a file.
Here is an example of opening a file in write mode.
#include<stdio.h>
void main( )
{
FILE *fp ;
char ch ;
fp = fopen(“file_handle.c”,”r”) ;
while ( 1 )
{
ch = fgetc ( fp ) ;
if ( ch == EOF )
break ;
printf(“%c”,ch) ;
}
fclose (fp ) ;
}
The output would be:
The content of the file will be printed.
#include;
void main( )
{
FILE *fp; // file pointer
char ch;
fp = fopen(“file_handle.c”,”r”);
while ( 1 )
{
ch = fgetc ( fp ); //Each character of the file is read and stored in the character file.
if ( ch == EOF )
break;
printf(“%c”,ch);
}
fclose (fp );
}
Closing File: fclose()
We use the fclose() function to close any given file. This file must be closed after we perform all the operations on it. Here is the syntax of the fclose() function:
int fclose( FILE *fp );
We hope this article was informative for you. Are you a GATE aspirant? Learn more on similar topics and Introduction to C Programming from here. So, prepare your best for the GATE exams, and crack it with flying colours this year. All the best!